Splash screen is the first screen of an app which open first when an app launch. It may represents the logo, name, or short description of an app for a short time. In this tutorial we will create a splash screen with jetpack compose.
For creating a Splash Screen we need two screens first for splash screen and second for Main Screen.
We can create two screens using composable Function or two separate Kotlin files for two screens.
The most important things that for navigation from Splash Screen to Main Screen we will use a compose navigation dependency in our build.gradle (Module:app) File.
implementation 'androidx.navigation:navigation-compose:2.5.3'
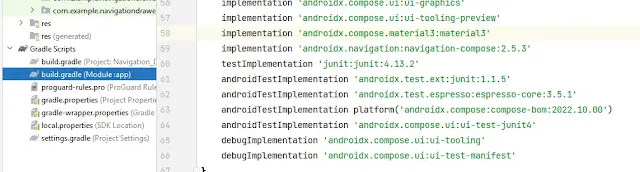
How to create Jetpack Compose Splash Screen?
Steps:
Lets Create a New Project named as Splash Screen Compose. Add an Image named as logo in drawable file. We will use this image in Splash Screen as logo with animation.
Create an Object file named “Screens” code and image is below.
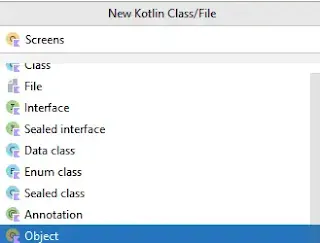
Create an object class Screens
Copy this code →
package com.example.splashscreencompose
object Screens {
const val Splash = "Splash"
const val Main = "MainScreen"
}
Now create two separate Kotlin Files for two screens.
Screen 1 is named as SplashScreen
Copy this code →
package com.example.splashscreencompose
import ...
@Composable
fun SplashScreen(navController: NavController)
= Box(
modifier = Modifier
.fillMaxWidth()
.fillMaxHeight())
{
val scale = remember {
androidx.compose.animation.core.Animatable(0.0f)
}
LaunchedEffect(key1 = true) {
scale.animateTo(
targetValue = 0.7f,
animationSpec = tween(800, easing = {
OvershootInterpolator(4f).getInterpolation(it)
}
)
)delay(1000)
//This stop to appear splash screen on pressing back button
navController.navigate(Screens.Main) {
popUpTo(Screens.Splash) {
inclusive = true
}
}
}
Image(painter = painterResource(id = R.drawable.logo),
contentDescription ="",
alignment = Alignment.Center, modifier = Modifier
.fillMaxSize().padding(40.dp)
.scale(scale.value)
)
Text(
text = "Version+${BuildConfig.VERSION_NAME}",
textAlign = TextAlign.Center,
fontSize = 24.sp,
modifier = Modifier.align(Alignment.BottomCenter).padding(16.dp)
)
}
Screen 2 named as MainScreen.
Copy this code →
package com.example.splashscreencompose
import ...
@Composable
fun MainScreen(navController = navController){
Text(text = "Main Screen", color = Color.Red, fontSize = 40.sp)
}
MainActivity
Copy this code →
package com.example.splashscreencompose
import ...
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
SplashScreenComposeTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
val navController = rememberNavController()
NavHost(
navController = navController,
startDestination = Screens.Splash
) {
composable(route = Screens.Splash) {
SplashScreen(navController = navController)
}
composable(route = Screens.Main) {
MainScreen(navController = navController)
}
}
}
}
}
}
}