How to build Bottom Sheet using Jetpack Compose
What is Bottom sheet ?
Material Design is an adaptable system of guidelines, components, and tools that support the best practices of user interface design. Backed by open-source code.
In this tutorial we will learn to create Bottom Sheet one of the most useful component in Material Design. It provides an easy way just like menu for displaying the components and menu option.It looks more stylish and attractive than Drop Down Menu that is similar in use.Bottom Sheet
Bottom sheets are surfaces containing supplementary content, anchored to the bottom of the screen just like bottom app bar but it animates from the bottom of the screen and it can hold more components than Bottom App bar.
Drag handle (optional)- The top 48dp portion of the bottom sheet is interactive when user-initiated resizing is available and the drag handle is present.
Read Also Splash Screen in Jetpack Compose
There are two types of bottom sheets:
1. Standard and2. Modal
Steps
All tutorials are based on Latest Version of Android Studio (Flamingo)
Create a New Project select Empty Activity.
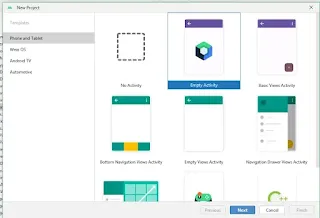
MainActivity
Copy this code →
package com.example.codingbihar
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.ui.Modifier
import com.example.codingbihar.ui.theme.CodingBiharTheme
import java.time.LocalDate
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
CodingBiharTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
BottomSheet()
}
}
}
}
Now create a separate new file for BottomSheet
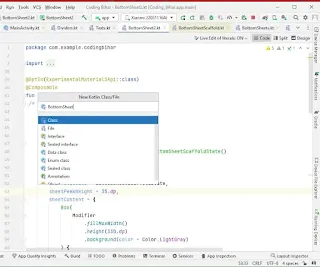
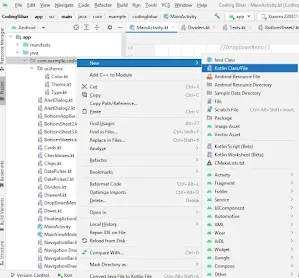
Bottom Sheet
Copy this code →
package com.example.codingbihar
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Add
import androidx.compose.material.icons.filled.Delete
import androidx.compose.material.icons.filled.Edit
import androidx.compose.material.icons.filled.Share
import androidx.compose.material3.BottomSheetScaffold
import androidx.compose.material3.Button
import androidx.compose.material3.ButtonDefaults
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.Icon
import androidx.compose.material3.Text
import androidx.compose.material3.rememberBottomSheetScaffoldState
import androidx.compose.runtime.Composable
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import kotlinx.coroutines.launch
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun BottomSheet() {
val bottomSheetScaffoldState = rememberBottomSheetScaffoldState()
val scope = rememberCoroutineScope()
BottomSheetScaffold(
scaffoldState = bottomSheetScaffoldState,
sheetPeekHeight = 35.dp,
sheetContent = {
Box(
Modifier
.fillMaxWidth()
.height(330.dp)
.background(color = Color.LightGray)
) {
Column(
Modifier.fillMaxSize(),
//verticalArrangement = Arrangement.Center,
//horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "codingbihar.com",
fontSize = 25.sp,
color = Color.Red,
fontWeight = FontWeight.ExtraBold
)
Text(
text = "Jetpack Compose",
fontSize = 18.sp,
color = Color.Black,
fontWeight = FontWeight.Bold
)
Text(text = "Bottom Sheet", fontSize = 22.sp, color = Color.Blue)
Row(Modifier.padding(top=12.dp)) {
Icon(imageVector = Icons.Filled.Share, contentDescription = "share",Modifier.padding(end=46.dp, start = 16.dp))
Icon(imageVector = Icons.Filled.Add, contentDescription = "Add",Modifier.padding(end=44.dp))
Icon(imageVector = Icons.Filled.Delete, contentDescription = "Delete",Modifier.padding(end=44.dp))
Icon(imageVector = Icons.Filled.Edit, contentDescription = "Edit",Modifier.padding(end=14.dp))
}
Row() {
Text(text = "Share",Modifier.padding(start = 10.dp))
Text(text = "Add",Modifier.padding(start = 22.dp))
Text(text = "Delete",Modifier.padding(start = 22.dp))
Text(text = "Edit",Modifier.padding(start = 22.dp))
}
}
}
},
){
}
Column(
Modifier.fillMaxSize(), verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally) {
Button(onClick = {
scope.launch {
bottomSheetScaffoldState.bottomSheetState.expand()
}
}, colors = ButtonDefaults.buttonColors(Color(0XFF0F9D58))) {
Text(text = "Swipe Up and Down", color = Color.White)
}
}
}
OUTPUT :
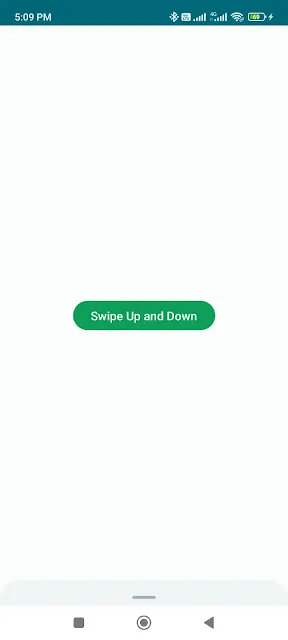
********************End*******
Material Design is an adaptable system of guidelines, components, and tools that support the best practices of user interface design. Backed by open-source code.
In this tutorial we will learn to create Bottom Sheet one of the most useful component in Material Design. It provides an easy way just like menu for displaying the components and menu option.
It looks more stylish and attractive than Drop Down Menu that is similar in use.
Bottom Sheet
Bottom sheets are surfaces containing supplementary content, anchored to the bottom of the screen just like bottom app bar but it animates from the bottom of the screen and it can hold more components than Bottom App bar.
Drag handle (optional)- The top 48dp portion of the bottom sheet is interactive when user-initiated resizing is available and the drag handle is present.
Read Also Splash Screen in Jetpack Compose
There are two types of bottom sheets:
1. Standard and
2. Modal
Steps
All tutorials are based on Latest Version of Android Studio (Flamingo)
Create a New Project select Empty Activity.
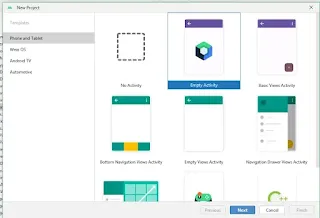
MainActivity
Copy this code →
package com.example.codingbihar
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.ui.Modifier
import com.example.codingbihar.ui.theme.CodingBiharTheme
import java.time.LocalDate
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
CodingBiharTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
BottomSheet()
}
}
}
}
Now create a separate new file for BottomSheet
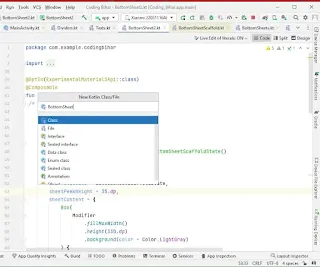
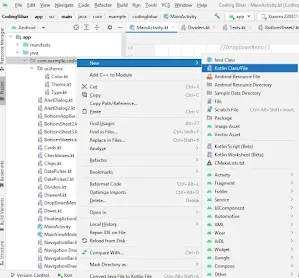
Bottom Sheet
Copy this code →
package com.example.codingbihar
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Add
import androidx.compose.material.icons.filled.Delete
import androidx.compose.material.icons.filled.Edit
import androidx.compose.material.icons.filled.Share
import androidx.compose.material3.BottomSheetScaffold
import androidx.compose.material3.Button
import androidx.compose.material3.ButtonDefaults
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.Icon
import androidx.compose.material3.Text
import androidx.compose.material3.rememberBottomSheetScaffoldState
import androidx.compose.runtime.Composable
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import kotlinx.coroutines.launch
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun BottomSheet() {
val bottomSheetScaffoldState = rememberBottomSheetScaffoldState()
val scope = rememberCoroutineScope()
BottomSheetScaffold(
scaffoldState = bottomSheetScaffoldState,
sheetPeekHeight = 35.dp,
sheetContent = {
Box(
Modifier
.fillMaxWidth()
.height(330.dp)
.background(color = Color.LightGray)
) {
Column(
Modifier.fillMaxSize(),
//verticalArrangement = Arrangement.Center,
//horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "codingbihar.com",
fontSize = 25.sp,
color = Color.Red,
fontWeight = FontWeight.ExtraBold
)
Text(
text = "Jetpack Compose",
fontSize = 18.sp,
color = Color.Black,
fontWeight = FontWeight.Bold
)
Text(text = "Bottom Sheet", fontSize = 22.sp, color = Color.Blue)
Row(Modifier.padding(top=12.dp)) {
Icon(imageVector = Icons.Filled.Share, contentDescription = "share",Modifier.padding(end=46.dp, start = 16.dp))
Icon(imageVector = Icons.Filled.Add, contentDescription = "Add",Modifier.padding(end=44.dp))
Icon(imageVector = Icons.Filled.Delete, contentDescription = "Delete",Modifier.padding(end=44.dp))
Icon(imageVector = Icons.Filled.Edit, contentDescription = "Edit",Modifier.padding(end=14.dp))
}
Row() {
Text(text = "Share",Modifier.padding(start = 10.dp))
Text(text = "Add",Modifier.padding(start = 22.dp))
Text(text = "Delete",Modifier.padding(start = 22.dp))
Text(text = "Edit",Modifier.padding(start = 22.dp))
}
}
}
},
){
}
Column(
Modifier.fillMaxSize(), verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally) {
Button(onClick = {
scope.launch {
bottomSheetScaffoldState.bottomSheetState.expand()
}
}, colors = ButtonDefaults.buttonColors(Color(0XFF0F9D58))) {
Text(text = "Swipe Up and Down", color = Color.White)
}
}
}
OUTPUT :
********************End*******
.png)