What is Alert Dialog
Alert Dialogs can be used to perform an action, communicate information, or help users accomplish a task. There are two types of dialogs:
1. Basic and
2. Full-screen
Android Alert Dialog is composed of three regions: title, content area and action buttons.
It can also be used to display the dialog message with OK and Cancel buttons. It can be used to interrupt and ask the user about his/her choice to continue or discontinue. Most of Android App uses Alert Dialog to confirm user to exit app.
In this tutorial we will learn to create a simple Alert Dialog and then we apply Alert Dialog on BackHandler.
Also Read Bottomsheet in Jetpack Compose
Steps :
Create a New Project and select Empty Activity.
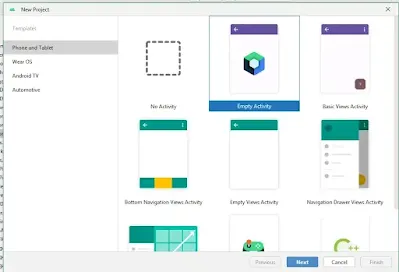
Simple Alert Dialog
Text
Copy this code →
package com.example.codingbihar
import androidx.compose.material3.AlertDialog
import androidx.compose.material3.Text
import androidx.compose.material3.TextButton
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
@Composable
fun AlertDialog() {
val openDialog = remember { mutableStateOf(true) }
if (openDialog.value) {
AlertDialog(
onDismissRequest = {
// Dismiss the dialog when the user clicks outside the dialog or on the back
// button. If you want to disable that functionality, simply use an empty
// onCloseRequest.
openDialog.value = false
},
title = {
Text(text = "Dialog")
},
text = {
Text(
"This is a simple Alert Dialog " +
"which presents the details regarding the Dialog's purpose."
)
},
confirmButton = {
TextButton(
onClick = {
openDialog.value = false
}
) {
Text("Confirm")
}
},
dismissButton = {
TextButton(
onClick = {
openDialog.value = false
}
) {
Text("Dismiss")
}
}
)
}
}
OUTPUT:

Now we will create an Alert Dialog and implements it on Back Handler so that when users click back button this Alert Dialog open if user select "Yes" they will exit from app and if choose "No" they will continue.
MainActivity
Copy this code →
package com.example.codingbihar
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.ui.Modifier
import com.example.codingbihar.ui.theme.CodingBiharTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
CodingBiharTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AlertDialog()
}
}
}
}
}
AlertDialog
Copy this code →
package com.example.codingbihar
import androidx.activity.compose.BackHandler
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.AlertDialog
import androidx.compose.material3.Button
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import kotlin.system.exitProcess
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun AlertDialog() {
var openDialog by remember { mutableStateOf(false) }
Text(text = "Android is open source available for developers," +
" designers and device makers." +
" That means we can experiment, imagine and create things that the world has never seen.")
BackHandler {
openDialog = true
}
if(openDialog){
AlertDialog(
onDismissRequest = {
openDialog = false
},
title = {
Text(text = "Jetapack Compose")
},
text = {
Text(
"This is a simple Alert Dialog." +
"DO you want to exit?"
)
},
confirmButton = {
Row(
modifier = Modifier.padding(all = 8.dp),
horizontalArrangement = Arrangement.Center
) {
Button(
modifier = Modifier.fillMaxWidth(),
onClick = { openDialog = false }
) {
Text("No")
}
}
},
dismissButton = {
Button(modifier = Modifier.fillMaxWidth(),
onClick = { val activity= MainActivity()
activity.finish()
exitProcess(0)
}
) {
Text(text = "Yes")
}
}
)
}
}
OUTPUT :



.png)